How to Write A Jenkinsfile
A Jenkinsfile is a text file that describes the entire build process for a Jenkins Pipeline. It uses the Groovy programming language and defines a Pipeline’s stages, steps, and conditions. Think of it as an instruction book on how to run your code. It is best practice to manage your Jenkinsfile within a source control system (such as git) alongside the codebase it is building. This allows developers to manage the build process alongside their code, making it easy to track changes over time.
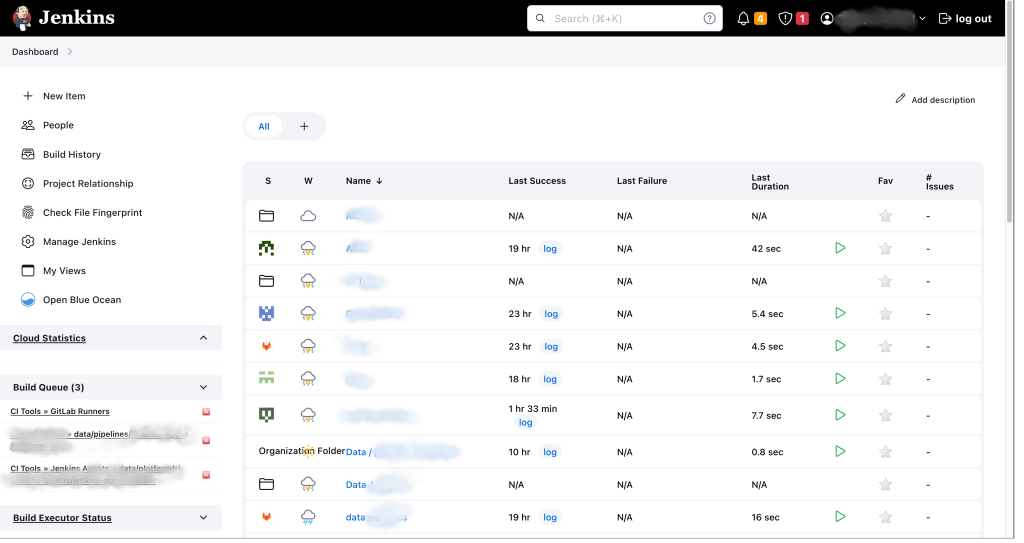
What information do you need to include in a Jenkinsfile?
A Jenkinsfile typically contains the following information:
- The pipeline syntax version.
- The agent that will execute the pipeline.
- The stages that make up the pipeline.
- The steps to be executed within each stage.
- Any pipeline options or settings, such as environment variables or custom parameters.
Jenkinsfile allows for more complex build and deployment processes, providing a powerful scripting language to define the entire build process. It also provides visibility into the build process, enabling developers to see the exact steps being taken and the status of each step.
Jenkinsfile Template
To write a Jenkinsfile, follow these steps:
Step 1 – Open / Create a Jenkinsfile in your favorite editor.
I prefer to use vs. code on Mac.
Open your favorite text editor and create a new file named “Jenkinsfile” in your project’s root directory.
Ensure the file is called Jenkinsfile, including the capital J. This is required and ensures that Jenkins will automatically pick up the Jenkinsfile when you push your code to source control.
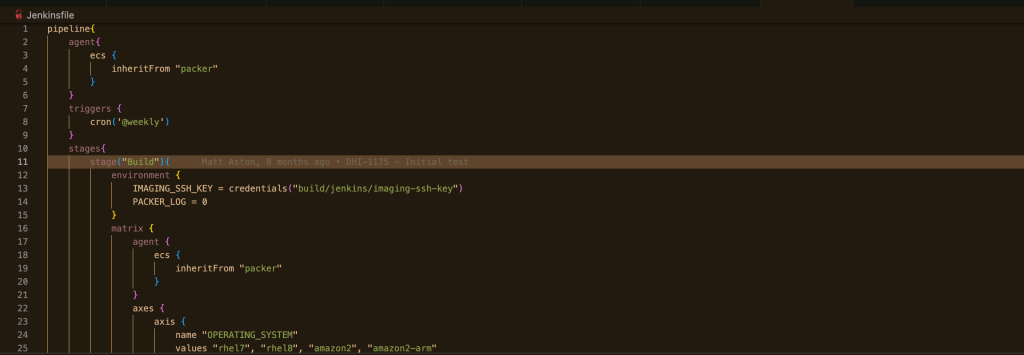
Step 2 – Define your Pipeline Agent
Begin by specifying the pipeline’s syntax version. The syntax version is necessary for Jenkins to understand your pipeline.
pipeline {
agent any
options {
// specify pipeline options here
}
For Example:
pipeline{
agent{
ecs {
inheritFrom "packer"
}
}
The Agent is where your Jenkins agent will execute. There are hundreds of different options available. Check out the official documentation here.
The agent section specifies the location where the build job will run. In this scenario, it’s on an ECS agent that is inheriting from a pre-configured Packer instance.
Overall, this script sets up a build environment using Jenkins, Packer, and ECS to create a reproducible and scalable build process.
Step 3 – Define your Stages
Next, specify the agent that will execute the pipeline. The agent specifies the environment in which the pipeline will run.
Define the stages of your pipeline. Each stage represents a specific step in your build process.
pipeline {
agent {
label 'my-agent'
}
stages {
stage('Build') {
steps {
// define build steps here
}
}
stage('Test') {
steps {
// define test steps here
}
}
stage('Deploy') {
steps {
// define deployment steps here
}
}
}
}
The stages
section defines the three stages of the build pipeline: Build, Test, and Deploy. Each stage contains a set steps
that defines the actions to be performed in that stage.
During the Build stage, you can define essential steps like compiling the code, packaging it into a distributable format, or performing other tasks to prepare the application for testing and deployment.
The Test stage involves defining the necessary steps to run application tests, including unit tests, integration tests, or other tests ensuring that the application functions as expected.
The Deploy stage is where you define essential steps for deploying the application to production environments, such as pushing the app to a server, updating database schemas, or performing other deployment steps to ensure smooth deployment
Step 4 – Define your Steps within each stage
Finally, define the steps within each stage. These steps will perform specific tasks, such as compiling code, running tests, or deploying to a server.
In this example, you will see a very basic setup to use each step of the terraform build process
pipeline {
agent any
stages {
stage('Plan') {
steps {
sh 'terraform init'
sh 'terraform plan -out=tfplan'
}
}
stage('Test') {
steps {
sh 'terraform validate'
}
}
stage('Apply') {
steps {
sh 'terraform apply -auto-approve tfplan'
}
}
}
}
Step 5 – Save and commit your Jenkinsfile
Save the Jenkinsfile in your project’s root directory.
Commit the Jenkinsfile to your version control system.
In Jenkins, create a new pipeline and specify the path to your Jenkinsfile.
Save the pipeline and run it to test your pipeline.
Jenkinsfile Template that uses source control and Terraform
Here is a basic example Jenkinsfile that connects to Bitbucket, downloads source code, and uses Terraform to deploy infrastructure:
pipeline {
agent any
environment {
TF_VERSION = "1.3.6"
}
stages {
stage('Checkout') {
steps {
checkout([$class: 'GitSCM',
branches: [[name: '*/main']],
doGenerateSubmoduleConfigurations: false,
extensions: [[$class: 'RelativeTargetDirectory', relativeTargetDir: 'my-app']],
submoduleCfg: [],
userRemoteConfigs: [[
url: '[email protected]:my-team/my-repo.git',
credentialsId: 'my-ssh-credentials'
]]
])
}
}
stage('Install Terraform') {
steps {
sh "wget https://releases.hashicorp.com/terraform/${TF_VERSION}/terraform_${TF_VERSION}_linux_amd64.zip -O terraform.zip"
sh "unzip terraform.zip"
sh "rm terraform.zip"
sh "mv terraform /usr/local/bin/"
}
}
stage('Deploy Infrastructure') {
steps {
dir('my-app/terraform') {
withCredentials([sshUserPrivateKey(credentialsId: 'my-ssh-credentials', keyFileVariable: 'SSH_KEY')]) {
sh """
ssh-agent bash -c 'ssh-add ${SSH_KEY}; terraform init'
ssh-agent bash -c 'ssh-add ${SSH_KEY}; terraform plan -out=tfplan'
ssh-agent bash -c 'ssh-add ${SSH_KEY}; terraform apply -auto-approve tfplan'
"""
}
}
}
}
}
}
1 Response
[…] In its simplest form, Terraform can be used to automatically create server infrastructure on several different platforms. It can also be integrated into Continuous Integration and Continuous Delivery (CICD). […]