PowerShell OneLiners for Active Directory
Active Directory is the backbone of many modern enterprise environmentsโa centralized platform for managing user accounts, groups, computers, and other resources. With PowerShell OneLiners, managing AD has never been more accessible. PowerShell is a powerful command-line tool that can help automate many tasks related to AD management.
This blog post will cover some of the most useful PowerShell oneliners for effective AD management.
You may want to create some random users to test these commands out. Here is a oneliner to create 20 random users:
1..20 | ForEach-Object { New-ADUser -Name ("User" + $_) -SamAccountName ("User" + $_) -AccountPassword (ConvertTo-SecureString "Password123!" -AsPlainText -Force) -Enabled $true -EmailAddress ("User" + $_ + "@turbogeek.co.uk") -GivenName (Get-Random -InputObject @("Alice", "Bob", "Charlie", "David", "Eva", "Frank", "Grace", "Henry", "Iris", "Jack")) -Surname (Get-Random -InputObject @("Smith", "Johnson", "Brown", "Lee", "Garcia", "Martinez", "Davis", "Taylor", "Wilson", "Anderson")) -StreetAddress (Get-Random -InputObject @("123 Main St.", "456 Elm St.", "789 Oak Ave.", "321 Pine St.", "654 Maple Ave.")) -City (Get-Random -InputObject @("New York", "Los Angeles", "Chicago", "Houston", "Philadelphia", "Phoenix", "San Antonio", "San Diego", "Dallas", "San Jose")) -State (Get-Random -InputObject @("CA", "TX", "NY", "FL", "IL", "PA", "OH", "GA", "NC", "MI")) -PostalCode (Get-Random -InputObject @(10001..99999)) }
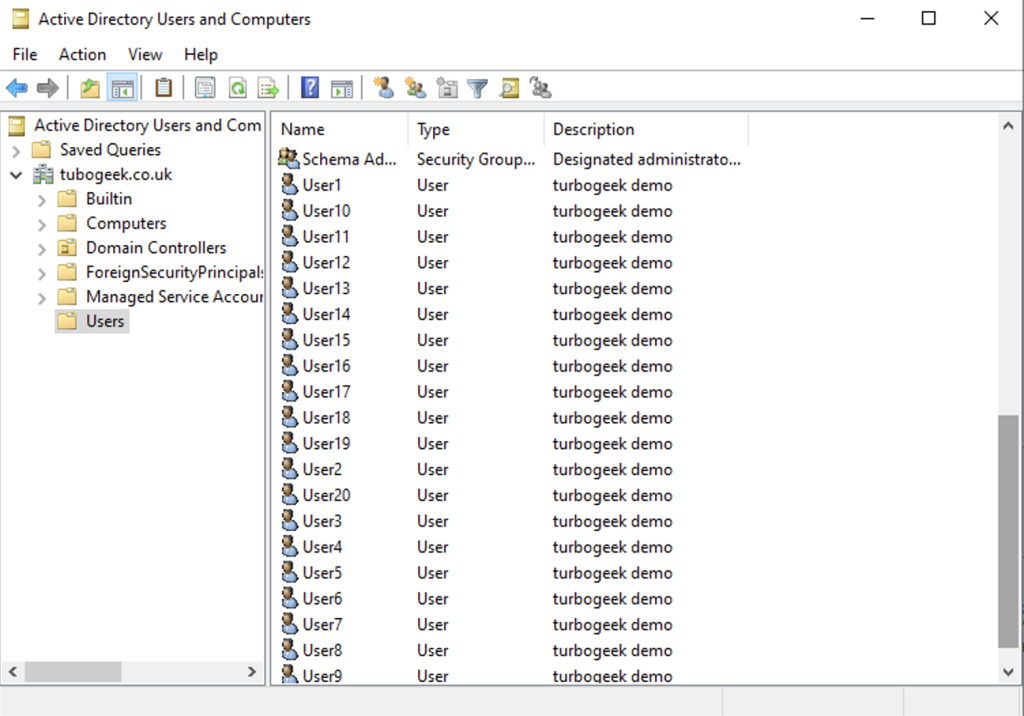
Get-ADUser
PowerShell One-Liners for Active Directory: The Basics
Get-ADUser is a PowerShell cmdlet that retrieves user accounts from AD. This cmdlet can search for user accounts based on various criteria such as username, email, department, etc. Here are some examples:
Retrieve all user accounts in the domain
Get-ADUser -Filter *
The PowerShell command Get-ADUser -Filter *
is used to retrieve information about Active Directory (AD) users. Let me break it down for you:
Get-ADUser
: This is the cmdlet (command-let) used in PowerShell to fetch information about Active Directory users.-Filter *
: This part of the command specifies the filter to be applied. In this case, the asterisk (*) is a wildcard character, and using it as the filter means that you want to retrieve all user objects without any specific conditions.
So, in simpler terms, the command is asking Active Directory to give you information about all its users. It’s a way to fetch a comprehensive list of user details without specifying any particular criteria for selection.
Get all user accounts in the Marketing department.
Get-ADUser -Filter 'Department -eq "Marketing"'
The PowerShell command Get-ADUser -Filter 'Department -eq "Marketing"'
is designed to retrieve information about Active Directory users who belong to the “Marketing” department. Let me break it down for you:
Get-ADUser
: This is the PowerShell cmdlet used to fetch information about Active Directory users.-Filter 'Department -eq "Marketing"'
: This part of the command is specifying a filter to narrow down the search. It is looking for users where the “Department” attribute is equal to “Marketing.”
So, in simpler terms, this command is telling Active Directory to provide details about users who are specifically part of the “Marketing” department. It helps you target and retrieve information about users based on a specific criterion, in this case, their department affiliation.
Recover a specific user account by username.
Get-ADUser -Identity "jdoe"
The PowerShell command Get-ADUser -Identity "jdoe"
is used to retrieve information about a specific Active Directory user with the username “jdoe.” Let me explain it in simpler terms:
Get-ADUser
: This is the PowerShell cmdlet used to fetch information about Active Directory users.-Identity "jdoe"
: This part of the command specifies the unique identifier of the user you want information about. In this case, it’s looking for the user with the username “jdoe.”
So, in simpler terms, this command is telling Active Directory to provide detailed information about the user whose username is “jdoe.” It allows you to target and retrieve specific details about a particular user in the Active Directory.
Get-ADUser is a powerful cmdlet that can help you quickly retrieve user accounts from Active Directory.
PowerShell One-Liners for Active Directory: Complex Oneliners
Retrieve all users who have not logged in for the past 90 days
Get-ADUser -Filter {LastLogonTimeStamp -lt (Get-Date).AddDays(-90)} -Properties LastLogonTimeStamp | Select Name, SamAccountName, LastLogonTimeStamp | Sort-Object LastLogonTimeStamp
This PowerShell command, Get-ADUser -Filter {LastLogonTimeStamp -lt (Get-Date).AddDays(-90)} -Properties LastLogonTimeStamp | Select Name, SamAccountName, LastLogonTimeStamp | Sort-Object LastLogonTimeStamp
, performs the following actions:
Get-ADUser
: This is the cmdlet used to retrieve information about Active Directory users.-Filter {LastLogonTimeStamp -lt (Get-Date).AddDays(-90)}
: This part of the command applies a filter to fetch users whoseLastLogonTimeStamp
is older than 90 days from the current date. It helps identify users who haven’t logged in for the specified period.-Properties LastLogonTimeStamp
: This ensures that theLastLogonTimeStamp
property is included in the information retrieved. This property contains the timestamp of the user’s last logon.Select Name, SamAccountName, LastLogonTimeStamp
: This part selects and displays specific properties for each user, namely their name, SamAccountName (username), and LastLogonTimeStamp.Sort-Object LastLogonTimeStamp
: Finally, the results are sorted based on theLastLogonTimeStamp
in ascending order. This helps organize the output with the users who haven’t logged in for the longest time appearing first.
In simpler terms, this command is used to find and display Active Directory users who haven’t logged in for the past 90 days, showing their names, usernames, and the timestamp of their last logon. The list is then sorted with the users who have been inactive the longest appearing first
Get-ADComputer
Basic Commands
Get-ADComputer is a PowerShell cmdlet that retrieves computer accounts from A. This cmdlet can search for computer accounts based on criteria such as name, operating system, etc. Here are some examples:
Retrieve all computer accounts in the domain.
Get-ADComputer -
Discover all computer accounts running Windows 10
Get-ADComputer -Filter 'OperatingSystem -like "Windows 10*"'
Get a specific computer account by name
Get-ADComputer -Identity "computer01"
Get-ADComputer is a useful cmdlet for managing computer accounts in Active Directory.
New-ADUser
ADUser is a PowerShell cmdlet that creates a new user account in AD This cmdlet can specify various properties for the new user account, such as name, password, department, and more.
Here are some examples:
Example 1: Create a new user account with default settings
New-ADUser -Name "John Doe" -SamAccountName "jdoe" -AccountPassword (ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force)
The PowerShell command New-ADUser -Name "John Doe" -SamAccountName "jdoe" -AccountPassword (ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force)
is used to create a new user account in Active Directory. Let me break down the components for you:
New-ADUser
: This is the cmdlet used to create a new user account in Active Directory.-Name "John Doe"
: This part specifies the full name of the new user, in this case, “John Doe.”-SamAccountName "jdoe"
: This sets the SamAccountName or username for the new user, in this case, “jdoe.”-AccountPassword (ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force)
: This section sets the password for the new user. It converts the plain text password “P@ssw0rd” into a secure string format, ensuring a more secure storage of the password.
In simpler terms, this command is creating a new user in Active Directory named “John Doe” with the username “jdoe” and setting the account password to “P@ssw0rd.” It’s a way to automate the process of adding a new user to the Active Directory system.
Example 2: Create a new user account with custom settings
New-ADUser -Name "Jane Smith" -SamAccountName "jsmith" -AccountPassword (ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force) -Department "Marketing" -EmailAddress "[email protected]"
The PowerShell command New-ADUser -Name "Jane Smith" -SamAccountName "jsmith" -AccountPassword (ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force) -Department "Marketing" -EmailAddress "[email protected]"
is used to create a new user account in Active Directory with specific attributes. Let’s break it down:
New-ADUser
: This cmdlet is used to create a new user account in Active Directory.-Name "Jane Smith"
: Specifies the full name of the new user as “Jane Smith.”-SamAccountName "jsmith"
: Sets the SamAccountName or username for the new user as “jsmith.”-AccountPassword (ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force)
: Sets the password for the new user. It converts the plain text password “P@ssw0rd” into a secure string format for added security.-Department "Marketing"
: Assigns the new user to the “Marketing” department.-EmailAddress "[email protected]"
: Specifies the email address for the new user as “[email protected].”
In simpler terms, this command creates a new user in Active Directory named “Jane Smith” with the username “jsmith.” The user is assigned to the “Marketing” department, and the account password is set to “P@ssw0rd.” Additionally, the email address “[email protected]” is associated with this user account. It’s a way to automate the process of adding a new user to Active Directory with specific details.
New-ADUser is a powerful cmdlet that can quickly help you create new user accounts in Active Directory.
New-ADGroup
New-ADGroup is a PowerShell cmdlet that creates a new group in AD. This cmdlet can specify various properties for the new group, such as name, description, and more. Here are some examples:
Example 1: Create a new group with default settings
New-ADGroup -Name "Marketing"
The PowerShell command New-ADGroup -Name "Marketing"
is used to create a new security group in Active Directory with the name “Marketing.” Let me break it down for you:
New-ADGroup
: This cmdlet is used to create a new group in Active Directory, and it can be either a security or distribution group.-Name "Marketing"
: This part specifies the name of the new group, and in this case, it is “Marketing.”
In simpler terms, this command is creating a new security group in Active Directory named “Marketing.” Security groups are often used to manage access permissions to resources, allowing you to grant or deny access to certain files, folders, or other network resources based on group membership.
Example 2: Create a with custom settings
New-ADGroup -Name "Sales" -Description "Group for sales department"
The PowerShell command New-ADGroup -Name "Sales" -Description "Group for sales department"
is used to create a new security group in Active Directory named “Sales” with a specified description. Let me break down the components for you:
New-ADGroup
: This cmdlet is used to create a new group in Active Directory, and it can be either a security or distribution group.-Name "Sales"
: This part specifies the name of the new group, and in this case, it is “Sales.”-Description "Group for sales department"
: This sets a description for the new group, providing additional information about its purpose or function. In this case, it indicates that the group is for the sales department.
In simpler terms, this command creates a new security group in Active Directory named “Sales” and includes a description indicating that it is intended for the sales department. Security groups are often used to manage access permissions to resources, allowing you to grant or deny access based on group membership. The description helps provide context or information about the purpose of the group.
New-ADGroup is a useful cmdlet for creating new groups in Active Directory.
Add-ADGroupMember
Add-ADGroupMember is a PowerShell cmdlet that adds a member to an AD group. This cmdlet can add users or other groups as group members. Here are some examples:
Example 1: Add a user to a group
Add-ADGroupMember -Identity "Marketing" -Members "jdoe"
The PowerShell command Add-ADGroupMember -Identity "Marketing" -Members "jdoe"
is used to add a user with the username “jdoe” to the existing security group named “Marketing” in Active Directory. Let me break down the components for you:
Add-ADGroupMember
: This cmdlet is used to add members to an Active Directory group.-Identity "Marketing"
: This specifies the group to which you want to add a member, in this case, “Marketing.”-Members "jdoe"
: This indicates the user you want to add to the group, and in this case, it is the user with the username “jdoe.”
In simpler terms, this command adds the user “jdoe” to the existing security group “Marketing” in Active Directory. Being a member of this group can grant specific permissions or access rights to resources assigned to the “Marketing” group. It’s a way to manage and control access for users within an organization.
Example 2: Add a group to another group
Add-ADGroupMember -Identity "Sales" -Members "Marketing"
The PowerShell command Add-ADGroupMember -Identity "Sales" -Members "Marketing"
is used to add a group named “Marketing” as a member of the existing security group “Sales” in Active Directory. Let me break down the components for you:
Add-ADGroupMember
: This cmdlet is used to add members to an Active Directory group.-Identity "Sales"
: This specifies the group to which you want to add a member, in this case, “Sales.”-Members "Marketing"
: This indicates the group you want to add to the “Sales” group, and in this case, it is the “Marketing” group.
In simpler terms, this command adds the entire “Marketing” group as a member of the existing security group “Sales” in Active Directory. This association allows members of the “Marketing” group to inherit the permissions or access rights granted to the “Sales” group. It’s a way to manage and organize access control within Active Directory by nesting groups.
Add-ADGroupMember is a useful cmdlet for managing group membership in Active Directory.
Remove-ADGroupMember
Remove-ADGroupMember is a PowerShell cmdlet that removes a member from an AD group. This cmdlet can remove users or other groups as group members. Here are some examples:
Example 1: Remove a user from a group
Remove-ADGroupMember -Identity "Marketing" -Members "jdoe"
The PowerShell command Remove-ADGroupMember -Identity "Marketing" -Members "jdoe"
is used to remove a user with the username “jdoe” from the security group named “Marketing” in Active Directory. Let’s break down the components:
Remove-ADGroupMember
: This cmdlet is used to remove members from an Active Directory group.-Identity "Marketing"
: This specifies the group from which you want to remove a member, in this case, “Marketing.”-Members "jdoe"
: This indicates the user you want to remove from the group, and in this case, it is the user with the username “jdoe.”
In simpler terms, this command removes the user “jdoe” from the security group “Marketing” in Active Directory. This action will revoke any permissions or access rights that were granted to the user based on their membership in the “Marketing” group. It’s a way to manage and control access for users within an organization by adjusting group memberships.
Example 2: Remove a group from another group
Remove-ADGroupMember -Identity "Sales" -Members "Marketing"
he PowerShell command Remove-ADGroupMember -Identity "Sales" -Members "Marketing"
is used to remove a group named “Marketing” from the membership of the existing security group “Sales” in Active Directory. Let me break down the components for you:
Remove-ADGroupMember
: This cmdlet is used to remove members from an Active Directory group.-Identity "Sales"
: This specifies the group from which you want to remove a member, in this case, “Sales.”-Members "Marketing"
: This indicates the group you want to remove from the “Sales” group, and in this case, it is the “Marketing” group.
In simpler terms, this command removes the entire “Marketing” group from the membership of the existing security group “Sales” in Active Directory. Any permissions or access rights granted to the “Marketing” group within the “Sales” group will be revoked. This is a way to manage and adjust group memberships for effective access control in Active Directory.
Remove-ADGroupMember is a useful cmdlet for managing group membership in Active Directory.
Set-ADUser
Set-ADUser is a PowerShell cmdlet that modifies the properties of an existing user account in Active Directory. This cmdlet can change various properties such as name, department, email, etc. Here are some examples:
Example 1: Change the department of a user
Set-ADUser -Identity "jdoe" -Department "Sales"
The PowerShell command Set-ADUser -Identity "jdoe" -Department "Sales"
is used to update the “Department” attribute for a specific user in Active Directory. Let me break down the components for you:
Set-ADUser
: This cmdlet is used to modify the properties of an Active Directory user.-Identity "jdoe"
: This specifies the user whose information you want to update, and in this case, it is the user with the username “jdoe.”-Department "Sales"
: This sets the “Department” attribute for the user to “Sales.” The “Department” attribute is a commonly used attribute to categorize or associate users with specific departments within an organization.
In simpler terms, this command updates the information for the user “jdoe” in Active Directory, changing their department affiliation to “Sales.” It’s a way to manage and maintain accurate user information within the Active Directory environment.
Example 2: Change the email address of a user
Set-ADUser -Identity "jdoe" -EmailAddress "[email protected]"
The PowerShell command Set-ADUser -Identity "jdoe" -EmailAddress "[email protected]"
is used to update the email address attribute for a specific user in Active Directory. Let’s break down the components:
Set-ADUser
: This cmdlet is used to modify the properties of an Active Directory user.-Identity "jdoe"
: This specifies the user whose information you want to update, and in this case, it is the user with the username “jdoe.”-EmailAddress "[email protected]"
: This sets the email address attribute for the user to “[email protected].” The email address attribute is used to store the user’s email address within the Active Directory.
In simpler terms, this command updates the email address for the user “jdoe” in Active Directory to “[email protected].” It’s a way to ensure that the user’s contact information is accurate and up-to-date in the Active Directory environment.
Set-ADUser is a powerful cmdlet for modifying user accounts in Active Directory.
PowerShell is a powerful tool for managing Active Directory more efficiently and effectively. Using the cmdlets we have covered in this post, you can quickly retrieve user and computer accounts, create new user accounts and groups, manage group membership, and modify user account properties. With PowerShell, you can automate many routine tasks related to Active Directory management, saving time and reducing errors.
Further Examples of PowerShell Oneliners
List all users in a specific Active Directory group:
Get-ADGroupMember -Identity "GroupName" | Select-Object Name
Let’s break it down:
- Get-ADGroupMember: This cmdlet is used to retrieve the members of an Active Directory group. In your case, it’s looking for members of the group specified in the “-Identity” parameter.
- -Identity “GroupName”: This part specifies the group for which you want to retrieve the members. You would replace “GroupName” with the actual name of the Active Directory group you’re interested in.
- | (Pipeline Operator): This operator takes the output from the command on its left (in this case, the members of the specified group) and passes it as input to the command on its right.
- Select-Object Name: This part selects and displays only the “Name” property of the objects passed through the pipeline. In this context, it will display the names of the members of the specified Active Directory group.
Find all disabled user accounts in Active Directory:
Get-ADUser -Filter 'Enabled -eq $false' -Properties Name, SamAccountName
- Get-ADUser: This cmdlet is used to retrieve user accounts from Active Directory.
- -Filter ‘Enabled -eq $false’: This part filters the results based on the “Enabled” property. It’s looking for user accounts where the “Enabled” attribute is equal to
$false
, meaning disabled accounts. This helps in finding and listing disabled user accounts in Active Directory. - -Properties Name, SamAccountName: This parameter specifies which additional properties of the user accounts should be retrieved and displayed. In this case, it includes the “Name” and “SamAccountName” properties.
List all active users in a specific Organizational Unit (OU) in Active Directory:
Get-ADUser -Filter 'Enabled -eq $true' -SearchBase "OU=OUName,DC=DomainName,DC=com" | Select-Object Name, SamAccountName
- Get-ADUser: This cmdlet retrieves Active Directory user accounts based on the specified parameters.
- -Filter ‘Enabled -eq $true’: This part of the command filters the results to include only enabled user accounts, ensuring that the output focuses on active users.
- -SearchBase “OU=OUName,DC=DomainName,DC=com”: Specifies the Organizational Unit (OU) in Active Directory where the search for users is conducted. Replace “OUName” with the specific organizational unit name and adjust the domain accordingly.
- | Select-Object Name, SamAccountName: After retrieving the user accounts, this part of the command selects and displays specific attributes for each user. In this case, it shows the “Name” and “SamAccountName” of the users, providing essential information for identification.
Create a new Active Directory user account with a specified password:
This PowerShell command creates a new Active Directory user with the specified display name, User Principal Name, password, and enables the account. The user is placed in the designated organizational unit within the specified domain, streamlining the process of user creation and account management in Active Directory
New-ADUser -Name "UserName" -UserPrincipalName "[email protected]" -AccountPassword (ConvertTo-SecureString "Password123" -AsPlainText -Force) -Enabled $true -Path "OU=OUName,DC=DomainName,DC=com"
- New-ADUser: This cmdlet is used to create a new user in Active Directory.
- -Name “UserName”: Specifies the display name of the new user. Replace “UserName” with the desired name for the user.
- -UserPrincipalName “[email protected]“: Sets the User Principal Name (UPN) for the new user. The UPN is typically in the format of an email address and serves as the user’s logon name.
- -AccountPassword (ConvertTo-SecureString “Password123” -AsPlainText -Force): Sets the password for the new user. The “ConvertTo-SecureString” cmdlet converts the plain text password “Password123” into a secure string, enhancing security. Adjust the password accordingly.
- -Enabled $true: Enables the new user account upon creation. This ensures that the user can log in immediately.
- -Path “OU=OUName,DC=DomainName,DC=com”: Specifies the Organizational Unit (OU) where the new user account will be created. Replace “OUName” with the specific organizational unit name, and adjust the domain accordingly.
Set the account expiration date for an Active Directory user account:
This PowerShell command updates the account expiration date for a specific Active Directory user, allowing for effective management of user access based on the defined expiration timeline. Ensure to replace “UserName” with the actual username and provide the appropriate date for the account expiration.
Set-ADUser -Identity "UserName" -AccountExpirationDate "mm/dd/yyyy"
- Set-ADUser: This cmdlet is used to modify properties of an existing Active Directory user.
- -Identity “UserName”: Specifies the user account to be modified. Replace “UserName” with the actual username.
- -AccountExpirationDate “mm/dd/yyyy”: Sets the account expiration date for the specified user. Replace “mm/dd/yyyy” with the desired date in the month/day/year format.
Find all Active Directory user accounts that have not logged in within a limited number of days:
In summary, this PowerShell command efficiently searches for inactive user accounts in Active Directory, focusing on users only and specifying a time span (30 days in this example). The selected attributes make it easy to identify and manage inactive accounts based on their names and SamAccountNames. Adjust the parameters as needed for your specific use case.
Search-ADAccount -UsersOnly -AccountInactive -TimeSpan "30.00:00:00" | Select-Object Name, SamAccountName
- Search-ADAccount: This cmdlet is used to search for user accounts in Active Directory based on specified criteria.
- -UsersOnly: Specifies that the search should focus only on user accounts, excluding other types of accounts such as computers or groups.
- -AccountInactive: Specifies that the search should include inactive accounts. This helps identify user accounts that have not been used within a specified timeframe.
- -TimeSpan “30.00:00:00”: Sets the time span for identifying inactive accounts. In this case, it looks for accounts that have been inactive for the past 30 days. Adjust the TimeSpan parameter as needed for your specific requirements.
- | Select-Object Name, SamAccountName: After identifying inactive user accounts, this part of the command selects and displays specific attributes for each user. In this case, it shows the “Name” and “SamAccountName” of the inactive users, providing essential information for further analysis or management.
Enable a disabled Active Directory user account:
Enable-ADAccount -Identity "UserName"
The PowerShell command Enable-ADAccount -Identity "UserName"
is used to enable or activate a disabled user account in Active Directory. Let’s break down the components:
Enable-ADAccount
: This cmdlet is used to enable a disabled user account in Active Directory.-Identity "UserName"
: This specifies the user account that you want to enable. In this case, it is referencing a user with the username “UserName.” Replace “UserName” with the actual username you want to enable.
In simpler terms, this command activates or enables the user account with the specified username (“UserName”) in Active Directory. If a user account was previously disabled, running this command will allow the user to log in and access resources again.
Reset the password for an Active Directory user account:
Set-ADAccountPassword -Identity "UserName" -NewPassword (ConvertTo-SecureString "NewPassword123" -AsPlainText -Force) -Reset
The PowerShell command Set-ADAccountPassword -Identity "UserName" -NewPassword (ConvertTo-SecureString "NewPassword123" -AsPlainText -Force) -Reset
is used to reset the password for a specified user account in Active Directory. Let’s break down the components:
Set-ADAccountPassword
: This cmdlet is used to set or reset the password for an Active Directory user account.-Identity "UserName"
: This specifies the user account for which you want to reset the password. Replace “UserName” with the actual username.-NewPassword (ConvertTo-SecureString "NewPassword123" -AsPlainText -Force)
: This sets the new password for the user account. The password “NewPassword123” is converted to a secure string format for enhanced security.-Reset
: This parameter indicates that the password is being reset. It is required when changing the password.
In simpler terms, this command resets the password for the specified user account (“UserName”) in Active Directory to “NewPassword123”. It’s a way to ensure security by periodically changing or resetting user passwords, and the secure string conversion helps protect the password during the process.
Find all Active Directory groups that a specific user is a member of:
Get-ADPrincipalGroupMembership -Identity "UserName" | Select-Object Name
The PowerShell command Get-ADPrincipalGroupMembership -Identity "UserName" | Select-Object Name
is used to retrieve the group memberships of a specified user in Active Directory and then selectively display the names of those groups. Let’s break down the components:
Get-ADPrincipalGroupMembership
: This cmdlet is used to retrieve the group memberships of a user or another principal in Active Directory.-Identity "UserName"
: This specifies the user for whom you want to retrieve group memberships. Replace “UserName” with the actual username.| Select-Object Name
: This part of the command selects and displays the names of the groups to which the user belongs.
In simpler terms, this command fetches and displays the names of the groups that the specified user (“UserName”) is a member of in Active Directory. It helps you identify the group memberships of a user for access control or administrative purposes.
Remove a user from an Active Directory group:
Remove-ADGroupMember -Identity "GroupName" -Members "UserName"
The PowerShell command Remove-ADGroupMember -Identity "GroupName" -Members "UserName"
is used to remove a specified user from a particular group in Active Directory. Let’s break down the components:
Remove-ADGroupMember
: This cmdlet is used to remove members from an Active Directory group.-Identity "GroupName"
: This specifies the group from which you want to remove a member. Replace “GroupName” with the actual name of the group.-Members "UserName"
: This indicates the user you want to remove from the group. Replace “UserName” with the actual username.
In simpler terms, this command removes the specified user (“UserName”) from the specified group (“GroupName”) in Active Directory. It’s a way to manage and control group memberships, adjusting access permissions for users within an organization.
Resetting passwords for multiple users in one line:
Get-ADUser -Filter * | ForEach-Object {Set-ADAccountPassword $_ -NewPassword (ConvertTo-SecureString "Password1234!" -AsPlainText -Force)}
The PowerShell command Get-ADUser -Filter * | ForEach-Object {Set-ADAccountPassword $_ -NewPassword (ConvertTo-SecureString "Password1234!" -AsPlainText -Force)}
is used to set a new password for all user accounts in Active Directory. Let’s break down the components:
Get-ADUser -Filter *
: This part of the command retrieves all user accounts in Active Directory without applying any specific filter (the wildcard ‘*’ means all users).| ForEach-Object {Set-ADAccountPassword $_ -NewPassword (ConvertTo-SecureString "Password1234!" -AsPlainText -Force)}
: This section uses a ForEach-Object loop to iterate through each user retrieved by the previous command. For each user, it sets a new password using theSet-ADAccountPassword
cmdlet.$_
: Represents the current user in the iteration.-NewPassword (ConvertTo-SecureString "Password1234!" -AsPlainText -Force)
: Specifies the new password for each user. The password “Password1234!” is converted to a secure string format for enhanced security.
In simpler terms, this command changes the password for all user accounts in Active Directory to “Password1234!”. This is a hypothetical example, and in a real-world scenario, you would likely want to implement more secure and complex password policies. Always ensure compliance with security best practices when managing passwords in an enterprise environment.
This will get all AD users and set their password to “Password1234!”.
Finding all users who haven’t changed their password in a while:
Get-ADUser -Filter * -Properties PasswordLastSet, PasswordNeverExpires | Where-Object {$_.PasswordLastSet -lt (Get-Date).AddDays(-90) -and $_.Enabled -eq $True -and $_.PasswordNeverExpires -eq $False} | Select-Object Name, SamAccountName, PasswordLastSet
This will find all users whose password was last set more than 90 days ago, are currently enabled, and whose password doesn’t ever expire. It will also display their name, account name, and the date their password was last set.
Creating a new user with multiple attributes set:
New-ADUser -Name "John Smith" -GivenName "John" -Surname "Smith" -SamAccountName "jsmith" -UserPrincipalName "[email protected]" -AccountPassword (ConvertTo-SecureString "Password1234!" -AsPlainText -Force) -Enabled $True -Path "OU=Users,OU=Contoso,DC=Contoso,DC=com"
This will create a new user named “John Smith” with the account name “jsmith”. It will set their password to “Password1234!” and enable the account. The user will be created in the “Users” OU within the “Contoso” domain.
Removing all members from a group except for a specified user:
Get-ADGroupMember -Identity "GroupName" | Where-Object {$_.SamAccountName -ne "Username"} | ForEach-Object {Remove-ADGroupMember -Identity "GroupName" -Members $_ -Confirm:$False}
This will get all members of a group named “GroupName” and remove all of them except for the user named “Username”.
Note: These oneliners can be pretty powerful and potentially destructive if misused. It’s essential to test them thoroughly in a non-production environment before running them in a production environment.
FAQ on PowerShell OneLiners for Active Directory
What are PowerShell One-Liners?
PowerShell One-Liners are commands that can be run in a single line of code in PowerShell. They are often used to perform quick tasks or to automate repetitive tasks in Active Directory.
Why should I use PowerShell OneLiners for Active Directory?
PowerShell One-Liners can save you time and effort when managing Active Directory. With just one line of code, you can perform tasks that would otherwise take multiple clicks or commands.
Can I use PowerShell OneLiners to manage multiple Active Directory domains?
Yes, PowerShell One-Liners can be used to manage multiple Active Directory domains. When running the command, you just need to specify the appropriate domain controller or forest.
What permissions do I need to run PowerShell OneLiners for Active Directory?
You need to have the appropriate permissions to manage Active Directory objects to run PowerShell One-Liners. This usually means being a member of the Domain Admins group or having delegated permissions.
Can I use PowerShell OneLiners to create new Active Directory objects?
Yes, PowerShell One-Liners can be used to create new Active Directory objects, such as user accounts, group accounts, and computer accounts.
How do I find specific Active Directory objects using PowerShell OneLiners?
You can use PowerShell One-Liners to search for specific Active Directory objects based on criteria such as name, description, or group membership. The Get-ADObject and Get-ADUser commands are often used for this purpose.
Can I use PowerShell OneLiners to modify existing Active Directory objects?
Yes, PowerShell One-Liners can be used to modify existing Active Directory objects, such as changing a user’s group membership or resetting a user’s password.
How do I delete Active Directory objects using PowerShell OneLiners?
You can use PowerShell One-Liners to delete Active Directory objects, such as user accounts or group accounts, using commands such as Remove-ADUser and Remove-ADGroup.
Can I use PowerShell OneLiners to generate reports or export data from Active Directory?
Yes, PowerShell One-Liners can be used to generate reports or export data from Active Directory. The Get-ADUser, Get-ADGroup, and Get-ADComputer commands can be used to retrieve information about Active Directory objects, which can then be formatted and exported using other PowerShell commands.
Where can I find examples of PowerShell OneLiners for Active Directory?
You can find examples of PowerShell One-Liners for Active Directory on various blogs and forums and in Microsoft’s official documentation. You can also create your own One-Liners to automate tasks specific to your environment.
2 Responses
[…] to get you started. All these steps can be completed using PowerShell; look out for the PowerShell […]
[…] Active Directory Oneliners […]