Sidestep the Pitfalls: 15 CDK Common Mistakes & How to Avoid Them
The AWS Cloud Development Kit (AWS CDK) is a framework for defining cloud resources in code and provisioning them through AWS CloudFormation. While it simplifies many aspects of defining cloud resources, there are still potential pitfalls or AWS-CDK Common Mistakes that users might encounter.
Here are some of the common mistakes:
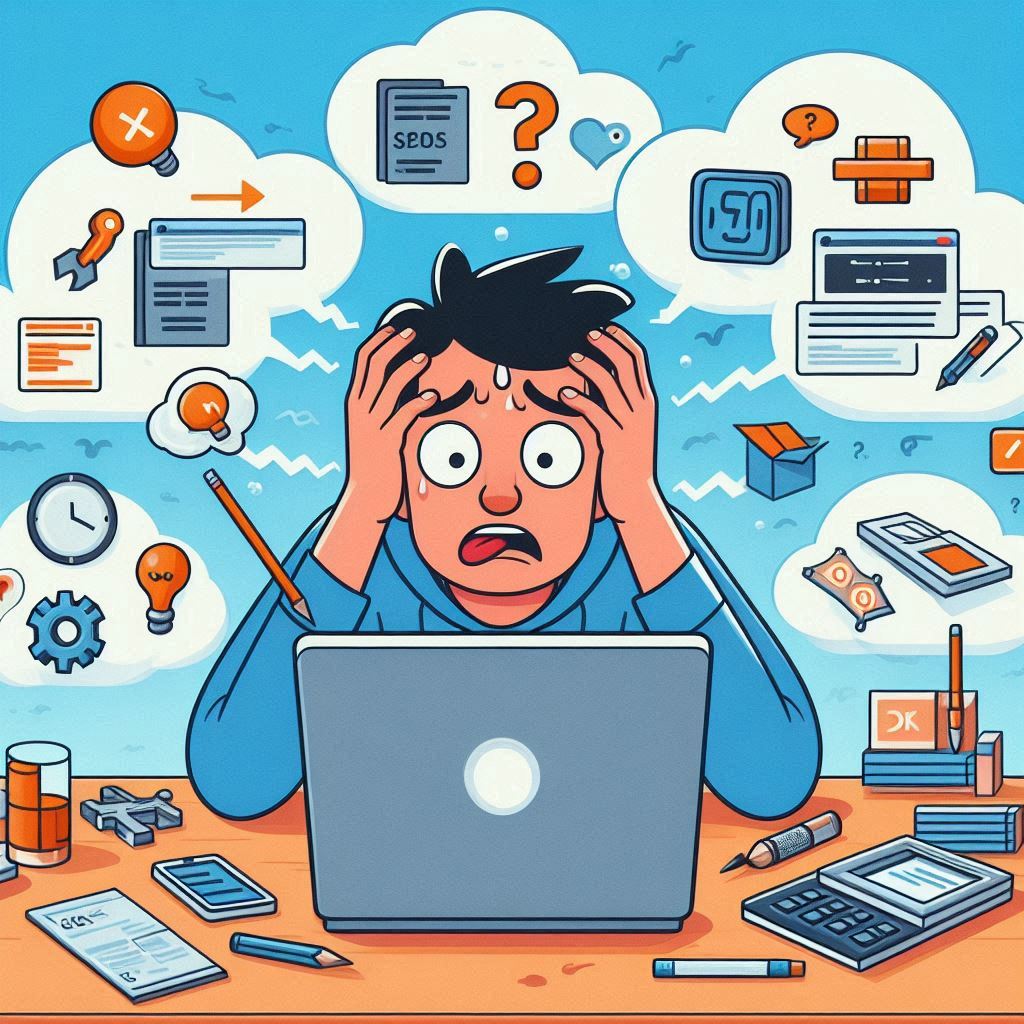
#1: Pinning CDK Versions:
- Given the rapid pace of development in CDK, there can be breaking changes between versions. It’s a good practice to pin the versions of CDK libraries in your
package.json
Or equivalent to ensure consistent behavior.
// Do this:
"dependencies": {
"@aws-cdk/core": "1.100.0"
}
#2: Imperative vs. Declarative:
- While CDK is more imperative than raw CloudFormation, you should still focus on describing “what” you want rather than “how” you get there.
#3: Hardcoding Values:
- Avoid hardcoding resource properties like ARNs, account IDs, or region names. Instead, use CDKโs mechanisms for parameterization.
// Instead of this:
const bucketArn = 'arn:aws:s3:::my-hardcoded-bucket-name';
// Do this:
const bucket = new s3.Bucket(this, 'MyBucket');
const bucketArn = bucket.bucketArn;
#4: Overuse of Default Permissions:
- The CDK has constructs that by default grant permissions that are too broad. Always review and restrict permissions to the least privilege necessary.
// Instead of this, which grants Lambda full S3 permissions:
const fn = new lambda.Function(this, 'MyFunction', {/* ... */});
const bucket = new s3.Bucket(this, 'MyBucket');
bucket.grantReadWrite(fn);
// Do this, which only grants the necessary permissions:
bucket.grantRead(fn);
#5: Failing to use Environment Agnostic Code:
- Not using
Stack.of(this).account
orStack.of(this).region
can make the code non-portable between accounts and regions.
// Instead of this:
const region = 'us-west-2';
// Do this:
const region = Stack.of(this).region;
#6: Not Handling Dependencies Explicitly:
- While CDK does a good job of figuring out dependencies between resources, there are situations where it’s necessary to define dependencies explicitly using the
addDependency
method.
const bucket = new s3.Bucket(this, 'MyBucket');
const fn = new lambda.Function(this, 'MyFunction', {
environment: {
BUCKET_NAME: bucket.bucketName
}
});
// Ensure Lambda has permissions and the bucket exists before deployment
fn.node.addDependency(bucket);
#7: Inefficient Loops:
- When using loops to create resources, be cautious. For instance, creating hundreds of resources in a loop might hit CloudFormation limits.
// Be cautious with loops like this:
for (let i = 0; i < 500; i++) {
new s3.Bucket(this, `Bucket${i}`);
}
#8: Forgetting to use Staging:
- Not taking advantage of CDK’s staging and environment capabilities can lead to potential issues when promoting code through different environments.
#9: Forgetting Cost Implications:
- Just because it’s easy to spin up resources with CDK doesnโt mean they are free. Always consider the costs associated with the AWS resources you are provisioning.
#10: Skipping Unit Tests:
- CDK code is still code. You can and should write unit tests, especially for more complex infrastructures.
// Sample jest test for a CDK stack
test('ECS Cluster Created', () => {
const app = new cdk.App();
const stack = new MyEcsStack(app, 'TestStack');
expect(stack).toHaveResource('AWS::ECS::Cluster');
});
#11: Not Reviewing Synthesized CloudFormation:
- While one of the benefits of the CDK is not having to write raw CloudFormation, it’s still a good practice to occasionally review the synthesized CloudFormation template to understand what’s being created.
#12: Ignoring Resource Limits:
- AWS services have various default and maximum limits. Be aware of these limits when designing and deploying resources.
// If creating many DynamoDB tables, be aware of account limits:
for (let i = 0; i < 300; i++) {
new dynamodb.Table(this, `Table${i}`, {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING }
});
}
#13: Not Keeping Up With CDK Updates:
- AWS often releases updates and bug fixes for the CDK. Regularly check for and apply updates.
#14: Forgetting Cleanup:
- Especially in development, you might create and test numerous stacks. Always remember to clean up to avoid unnecessary costs.
#15: Not Leveraging Existing Constructs:
- The CDK has a growing library of constructs (L1, L2, and L3). Before reinventing the wheel, check if there’s an existing construct that meets your needs.
// Instead of using L1 constructs (low-level):
new s3.CfnBucket(this, 'Bucket', {
bucketName: 'my-bucket'
});
// Use L2 constructs when available for additional features and ease:
new s3.Bucket(this, 'MyBucket', {
removalPolicy: cdk.RemovalPolicy.DESTROY
});
To avoid these and other AWS-CDK Common Mistakes, always read the AWS CDK documentation, use best practices, and test thoroughly before deploying to production environments.
The AWS CDK is a powerful tool for streamlining cloud development workflows. By understanding and avoiding these common mistakes, you’ll be well on your way to building robust, scalable, and cost-effective infrastructure.
1 Response
[…] can automate infrastructure provisioning and application deployment by leveraging AWS services like AWS CloudFormation and AWS OpsWorks, reducing human error and streamlining operations. Regular monitoring and logging […]