How Object-Oriented Programming Principles work in AWS CDK
The AWS Cloud Development Kit (CDK) has simplified how developers define and provision cloud infrastructure, transforming it from static configuration files into dynamic, programmable code.
I use TypeScript with CDK, a popular strongly-typed superset of JavaScript, the AWS CDK allows developers to use the principles of Object-Oriented Programming (OOP).
This article explores how the core principles of OOP—encapsulation, inheritance, polymorphism, and abstraction—manifest within AWS CDK TypeScript projects, enabling more robust, maintainable, and scalable infrastructure as code (IaC).
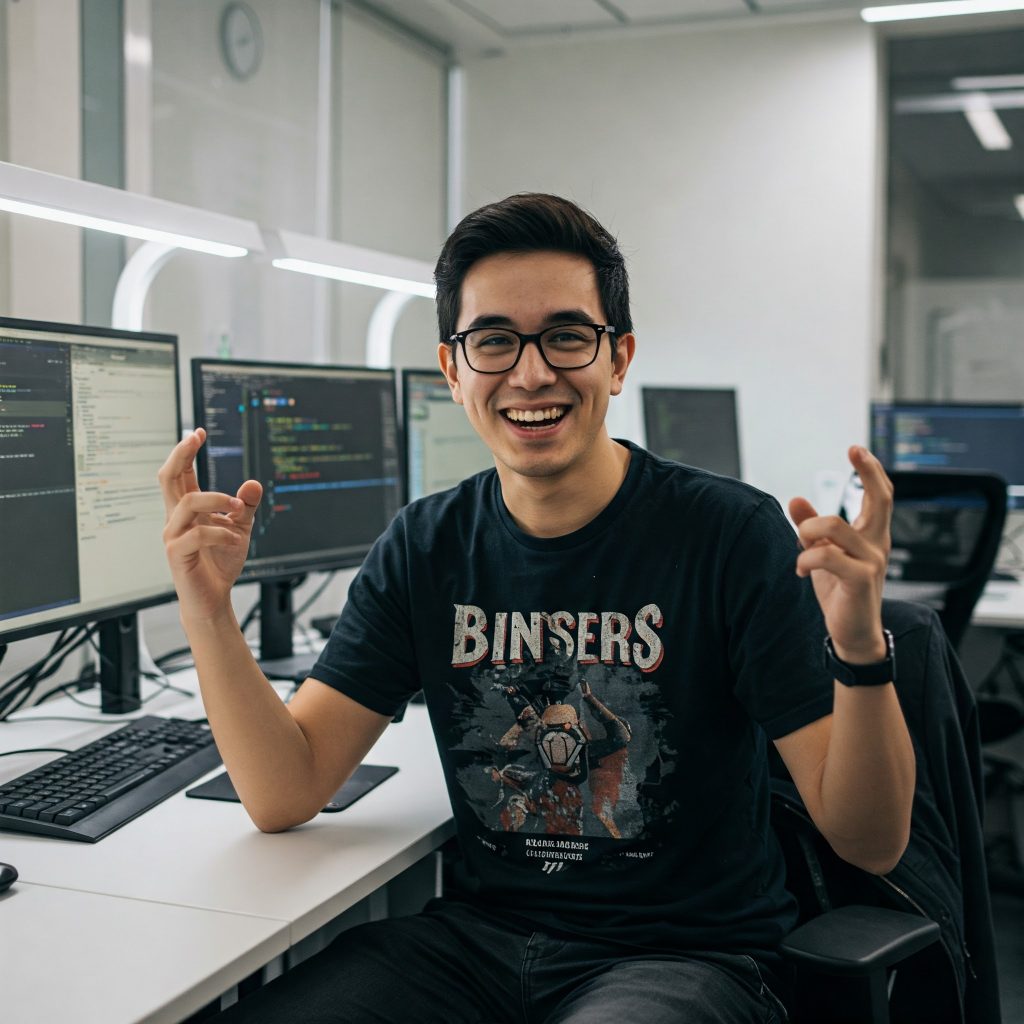
Understanding OOP in the Context of AWS CDK
Before diving into specifics, let’s briefly revisit the fundamental OOP principles:
Encapsulation:
Bundling data (properties) and the methods that operate on that data within a single unit (an object). This hides the internal state and complexity from the outside world, exposing only a well-defined interface.
Inheritance:
Allowing a new class (subclass or derived class) to acquire the properties and methods of an existing class (superclass or base class). This promotes code reuse and establishes a hierarchical relationship between classes.
Polymorphism:
Enabling objects of different classes to respond to the same message (method call) in different ways. This allows for more flexible and extensible systems.
Abstraction:
Simplifying complex reality by modeling classes based on relevant attributes and behaviors. It focuses on what an object does rather than how it does it, hiding unnecessary details.
In AWS CDK, these principles are not just theoretical concepts but are actively employed to structure, create, and manage AWS resources.
Encapsulation: Containing Complexity with Constructs
At the heart of AWS CDK are Constructs, which are the basic building blocks representing AWS resources or a collection of resources. Constructs inherently embody the principle of encapsulation.
- Hiding Internal Details: When you instantiate a CDK construct like
new s3.Bucket(this, 'MyBucket');
, you are interacting with a high-level object. The intricate details of how that S3 bucket is defined in AWS CloudFormation—its properties, dependencies, and lifecycle—are encapsulated within theBucket
class. You don’t need to manage the raw CloudFormation JSON; the construct handles it. - Well-Defined Interfaces: Constructs expose methods and properties to configure the resource (e.g.,
bucket.addLifecycleRule()
,bucket.grantRead()
). This interface is the approved way to interact with the resource’s configuration, preventing accidental misconfiguration of internal state. - State Management: The construct manages its own state. For instance, adding an event notification to an S3 bucket that triggers a Lambda function involves methods on both the
Bucket
andFunction
constructs. The CDK orchestrates the necessary permissions and connections, encapsulating this complexity.
This encapsulation makes CDK code easier to read, reason about, and maintain, as developers can work with higher-level resource objects rather than getting bogged down in low-level details.
Inheritance: Building and Customizing with Class Hierarchies
TypeScript’s support for classes and inheritance is a natural fit for AWS CDK, allowing developers to extend and customize existing constructs or create entirely new, reusable components.
- Extending Base Constructs: You can create your own custom construct by extending a base CDK class, such as
Construct
or a more specific L2 construct likes3.Bucket
. This allows you to add default configurations, helper methods, or composite resources tailored to your organization’s best practices.
import * as s3 from 'aws-cdk-lib/aws-s3';
import { Construct } from 'constructs';
import { RemovalPolicy } from 'aws-cdk-lib';
export interface SecureBucketProps extends s3.BucketProps {
customLoggingConfig?: object; // Example of an additional property
}
export class SecureBucket extends s3.Bucket {
constructor(scope: Construct, id: string, props?: SecureBucketProps) {
const defaultProps: Partial<s3.BucketProps> = {
encryption: s3.BucketEncryption.S3_MANAGED,
blockPublicAccess: s3.BlockPublicAccess.BLOCK_ALL,
versioned: true,
removalPolicy: RemovalPolicy.RETAIN, // Sensible default
...props, // User-provided props can override defaults
};
super(scope, id, defaultProps);
// Add custom logic, e.g., applying specific logging
if (props?.customLoggingConfig) {
// Apply customLoggingConfig
}
this.node.addValidation({
validate: () => {
const messages: string[] = [];
if (this.encryption !== s3.BucketEncryption.S3_MANAGED && this.encryption !== s3.BucketEncryption.KMS_MANAGED && this.encryption !== s3.BucketEncryption.KMS) {
messages.push('SecureBucket must have S3_MANAGED or KMS encryption.');
}
return messages;
}
});
}
// Custom method
public addSecureLifecycleRule(rule: s3.LifecycleRule): void {
// Potentially add validation or defaults before adding the rule
this.addLifecycleRule(rule);
}
}
- Creating Reusable Patterns (L3 Constructs/Patterns): Inheritance is fundamental to creating higher-level abstractions, often referred to as L3 constructs or patterns. These constructs can encapsulate entire application architectures (e.g., a load-balanced Fargate service) by composing and configuring multiple L1 and L2 constructs. By defining these patterns as classes, they can be easily instantiated and reused across multiple projects or stacks.
- Shared Functionality: Base classes can provide common functionality (e.g., tagging, naming conventions) that all derived custom constructs automatically inherit.
Polymorphism: Flexible Interactions with Diverse Constructs
Polymorphism in AWS CDK with TypeScript often appears through interfaces and method overriding, allowing for flexible and standardized interactions between different types of constructs.
- Interfaces as Contracts: TypeScript interfaces can define a contract that different constructs can implement. For example, you might define an
IBackupStrategy
interface with anapplyBackup(resource: ec2.Instance | rds.DatabaseInstance)
method. Different classes could implement this interface to provide specific backup logic for EC2 instances versus RDS databases, yet they could be treated uniformly by a backup management system.
import * as ec2 from 'aws-cdk-lib/aws-ec2';
import * as rds from 'aws-cdk-lib/aws-rds';
import { Construct } from 'constructs';
// Define an interface for resources that can be granted access
interface IGrantable {
grantRead(grantee: iam.IGrantee): void;
}
// S3 Bucket could implement IGrantable (it already does via s3.IBucket)
// A custom database construct could also implement IGrantable
class MyCustomDatabase extends Construct implements IGrantable {
// ... internal implementation ...
constructor(scope: Construct, id: string) {
super(scope, id);
// ... setup database resources ...
}
public grantRead(grantee: iam.IGrantee): void {
// ... logic to grant read access to this custom database ...
console.log(`Granting read access to ${grantee} for MyCustomDatabase ${this.node.id}`);
}
}
// Usage
const someRole = new iam.Role(/* ... */);
const s3Bucket = new s3.Bucket(/* ... */);
const customDb = new MyCustomDatabase(/* ... */);
const grantableResources: IGrantable[] = [s3Bucket, customDb];
grantableResources.forEach(resource => {
resource.grantRead(someRole); // Polymorphic call
});
- Method Overriding: When creating custom constructs by extending base classes, you can override methods to alter or specialize their behavior. For example, a custom
MyVpc
construct inheriting fromec2.Vpc
might override theaddGatewayEndpoint
method to automatically add certain tags or logging whenever a gateway endpoint is created. - Generic Functions and Classes: TypeScript’s generics can be used with CDK constructs to create functions or classes that operate on a variety of construct types while maintaining type safety. For example, a function could apply a standard set of tags to any construct that extends
cdk.Resource
.
While perhaps less explicit than in application programming, polymorphism in CDK enables the creation of generalized tooling and patterns that can operate over a diverse set of resources in a consistent manner.
Abstraction: Simplifying Complexity at Multiple Levels
Abstraction is a cornerstone of the AWS CDK’s design, simplifying the immense complexity of cloud infrastructure.
- Levels of Abstraction (L1, L2, L3):
- L1 (CFN Resources): These are low-level constructs that directly map one-to-one with AWS CloudFormation resources. They provide complete control but require understanding CloudFormation details. Example:
new CfnBucket(this, 'MyCfnBucket', { bucketName: 'my-cfn-bucket' });.
- L2 (Curated Constructs): These are higher-level constructs that represent AWS resources with a more intuitive, intent-based API. They provide sensible defaults, boilerplate reduction, and helper methods. This is the most common level for application developers. Example:
new s3.Bucket(this, 'MyL2Bucket', { versioned: true });.
- L3 (Patterns): These are even higher-level abstractions that encapsulate common architectural patterns, often involving multiple L2 constructs. Each level provides a different degree of abstraction, allowing developers to choose the level of detail they need to manage. Example:
new ecsPatterns.ApplicationLoadBalancedFargateService(this, 'MyFargateService', { /* ... */ });.
- Custom Abstractions: By creating your own L2 or L3 constructs, you build custom abstractions that are meaningful to your specific domain or organization. A
SecureCompanyApi
construct, for example, could abstract away the details of API Gateway, Lambda, WAF, and IAM roles, presenting a simple interface for developers to deploy a new secure API endpoint. - Focus on “What,” Not “How”: With higher-level constructs, you declare what resources you want and their desired state, rather than imperatively defining how to create and configure every individual piece. The CDK handles the “how” based on the abstractions provided.
OOP and IaC for Better Cloud Development
The application of object-oriented principles within the AWS CDK using TypeScript offers significant advantages:
- Reusability: Inheritance and composition allow for the creation of reusable constructs and patterns, reducing code duplication and ensuring consistency.
- Maintainability: Encapsulation and abstraction lead to cleaner, more organized code that is easier to understand, modify, and debug. Changes within a construct are less likely to impact other parts of the infrastructure.
- Scalability: Well-structured, object-oriented IaC can more easily scale to manage complex applications and environments.
- Developer Productivity: Familiar OOP concepts and the power of TypeScript (including type safety, autocompletion, and refactoring in IDEs) enhance the developer experience and speed up development.
- Testability: OOP design facilitates unit testing of custom constructs, ensuring that infrastructure components behave as expected before deployment.
By embracing these OOP principles, development teams can transform their infrastructure-as-code practices from writing declarative templates to building sophisticated, well-engineered cloud systems with the AWS CDK and TypeScript. This approach not only makes managing cloud resources more efficient but also aligns infrastructure development more closely with modern software engineering best practices.
Recent Comments